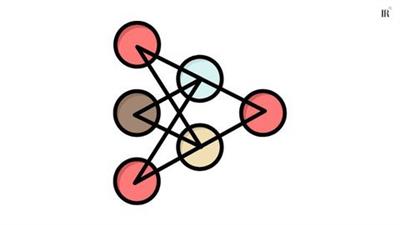
Programming Practices: Algorithms And Data Structures
Published 4/2023
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 277.36 MB | Duration: 0h 53m
Software practices, Algorithms, Data Structures, Software Engineering, Productivity, Clean Code
Published 4/2023
MP4 | Video: h264, 1280x720 | Audio: AAC, 44.1 KHz
Language: English | Size: 277.36 MB | Duration: 0h 53m
Software practices, Algorithms, Data Structures, Software Engineering, Productivity, Clean Code
What you'll learn
Basic concepts of Algorithms from programming practices perspective
Basic concepts of Data structures from programming practices perspective
A clear explanation of commonly used data strctures and algorithms independent of programming language
Decision framework to choose the optimal data strcuture and algorithm
Requirements
No programming experience needed. Familiarity with one or more programming languages would be definitely helpful to appreciate the generic knowledge.
Description
Welcome to Practical Algorithms and Data Structures for Programming, a one-hour course designed to help you understand and effectively use algorithms and data structures in your programming practice. This course covers a wide range of topics, from the basics of algorithms and data structures to more advanced concepts, with a focus on real-world programming scenarios.Note: The content of this course is also published as part of an in-depth course titled "Programming Practices Bootcamp for production ready coding."Course Outline:Course IntroductionOverview of algorithms and data structures in programming practicesData Structures: HashMapPrimary Purpose, Implementation Overview, ConsiderationsShould You Write Your Own Hash Function, ConclusionAlgorithms and Data Structures IntroductionOverview, Complex Data Structures, Understanding the BasicsSearch AlgorithmsLinear Complexity Concept, Linear Search ExampleUnderstanding the Language Libraries, Logarithmic ComplexitySorted Data Is Better for SearchSorting AlgorithmsIntroduction, Basic Concept, Implement and UnderstandPivot Vulnerability, Tuning to Handle Data PatternsAlgorithm Libraries, Comparison Functions, Return Types, and Error ConditionsAlgorithm Big O NotationNecessity, Small Data Behavior SurprisesData Structures: Dynamic ArraysIntroduction, Advantages, Maintaining Sorted CostReallocation, Deleting Elements, Moving DataData Structures: ListsIntroduction, Basic Concept, Storage StrategyReorganization Strategy, Validity After ModificationsWhen to Use Lists, Availability in Different LanguagesData Structures: TreesIntroduction, Types of Trees, CRUD OperationsTraversals, Real-World Applications of TreesBy the end of this course, you'll have a solid understanding of algorithms and data structures, and how to effectively use them in your programming practice. With practical examples and insights, this course will help you enhance your programming skills and build more efficient applications. Enroll now and start mastering algorithms and data structures for programming!
Overview
Section 1: Introduction
Lecture 1 Introduction
Section 2: Software Practices for programming with Algorithms and Data Structures
Lecture 2 Overview
Lecture 3 Data structure overview
Lecture 4 Complex Data Strcutures
Lecture 5 Importance of understanding the basics
Section 3: Algorithms and programming practices
Lecture 6 Search algorithms
Lecture 7 The concept of linear complexity in algorithms
Lecture 8 Example of linear search
Lecture 9 Algorithm implementations provided by the programming language libraries
Lecture 10 Algorithms and logarithmic complexity (coding interview favorite! )
Lecture 11 Why sorting is important for search algorithms?
Section 4: Sorting Algorithms
Lecture 12 Introduction
Lecture 13 Basic Concept of sorting algorithms from programming practices perspective
Lecture 14 Curcial to implement yourself to understand the basics well
Lecture 15 Quick sort and the pivot vulnerability
Lecture 16 Reality is more prone to data patterns than the coding interview tries to show
Section 5: Algorithms and Libraries
Lecture 17 Using libraries for algorithms is recommended instead or reinventing the wheel
Lecture 18 The role of comparison functions in algorithms and programming practices
Lecture 19 Return types and Error conditions related programming practices for algorithms
Section 6: The Big-O notation overview
Lecture 20 Algorithms and the famous Big O notation
Lecture 21 Necessity for seemigly confusion theoretical notation and procedure to calculate
Lecture 22 Theory is good but reality can pack some surprises.
Section 7: Dynamic Arrays Data Strcutures
Lecture 23 Basic concept of Dynamic Arrays
Lecture 24 Adavantages provided by dyanmics array data structures
Lecture 25 Sorting and dynamic arrays
Lecture 26 Resource management of growing dynamic arrays
Lecture 27 Deleteing Elements from a dynamic array
Lecture 28 Data movement and dynamic arrays
Section 8: List data structure
Lecture 29 Overview of List as a data structure
Lecture 30 Basic concept
Lecture 31 Storage Strategy used by List
Lecture 32 Reorganization strategy used by list
Lecture 33 Data validity post modification
Lecture 34 When to use list data structure?
Lecture 35 Does every language provide a built in list data structure?
Section 9: Tree data structure
Lecture 36 Overview
Lecture 37 Types of trees
Lecture 38 CRUD operations on trees
Lecture 39 Tree traversals for data access
Lecture 40 Who uses trees?
Section 10: Hashmap data structure
Lecture 41 Introduction
Lecture 42 Primary Purpose of hashmap data structures
Lecture 43 How are hashmap implemented? (pseudo code)
Lecture 44 Consideration while working with hashmaps
Lecture 45 Should one write their own hash functions everytime?
Section 11: Conclusion
Lecture 46 Closing remarks
Lecture 47onus Lecture
Beginner developers,Students who have recently learned any programming language,Programmers curious to understand the importance of algorithms and data strcutures in software engineering.
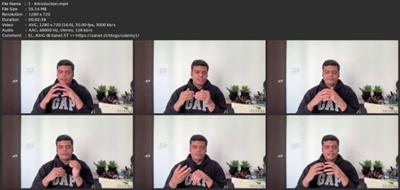
Download link
rapidgator.net:
Код:
https://rapidgator.net/file/4c487189c21ea57fba5ee7a755bbb113/oktqo.Programming.Practices.Algorithms.And.Data.Structures.rar.html
nitroflare.com:
Код:
https://nitroflare.com/view/E386F260BFEE0AE/oktqo.Programming.Practices.Algorithms.And.Data.Structures.rar
ddownload.com:
Код:
https://ddownload.com/b9i8tosl6vif/oktqo.Programming.Practices.Algorithms.And.Data.Structures.rar
1dl.net:
Код:
https://1dl.net/8f8pj3lj6sbh/oktqo.Programming.Practices.Algorithms.And.Data.Structures.rar